18. 作例2
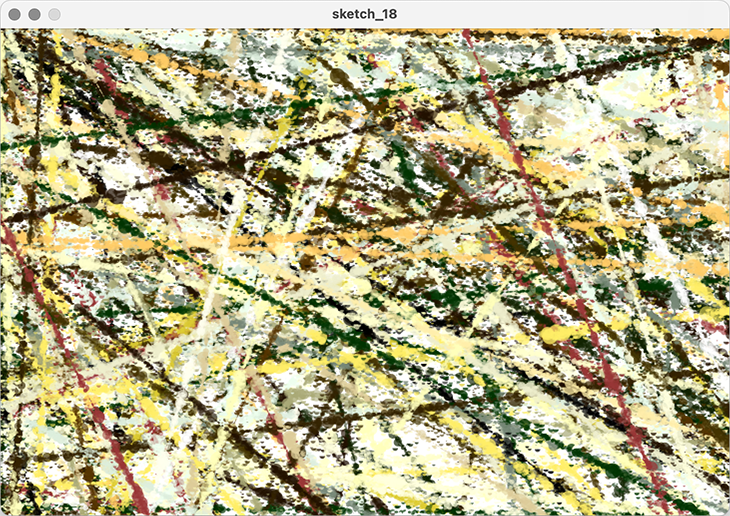
void setup() {
size( 730, 488 );
background( 255 );
smooth();
noLoop();
noStroke();
ellipseMode( RADIUS );
}
void draw() {
//配列や変数の定義
//色の配列24色を定義
color[] colorArray = { color( 0, 0, 0 ), color( 255, 255, 255 ),
color( 255, 255, 238 ), color( 255, 255, 221 ),
color( 255, 255, 204 ), color( 255, 255, 187 ),
color( 238, 238, 204 ), color( 255, 238, 170 ),
color( 221, 204, 153 ), color( 255, 238, 102 ),
color( 255, 204, 102 ), color( 170, 170, 119 ),
color( 221, 204, 51 ), color( 0, 51, 0 ),
color( 68, 51, 0 ), color( 85, 51, 0 ),
color( 51, 34, 0 ), color( 51, 34, 0 ),
color( 34, 17, 0 ), color( 17, 34, 0 ),
color( 238, 255, 238 ), color( 221, 238, 221 ),
color( 119, 136, 119 ), color( 153, 54, 54 ) };
int pointNum = 200; //座標の数
float[][] pointArray = new float[pointNum][2]; //座標用の配列
//描画単位の軌跡を決める座標を配列に格納
//画面の上端下端、左右を繰り返すように値を格納
for( int i = 0; i < pointNum; i++ ) {
if( i % 4 == 0 ) {
pointArray[i][0] = 1;
pointArray[i][1] = random( height );
} else if ( i % 4 == 1 ) {
pointArray[i][0] = width;
pointArray[i][1] = random( height );
} else if( i % 4 == 2 ) {
pointArray[i][0] = random( width );
pointArray[i][1] = 1;
} else if( i % 4 == 3 ) {
pointArray[i][0] = random( width );
pointArray[i][1] = height;
}
}
int baseSize = 12; //基本になる描画単位のサイズ
float shapeSize = 0;
float shapePitch = 0; //調整後の描画単位の間隔
float angle = 0; //軌跡の角度
float plusX = 0; //軌跡に添うようなxの増分
float plusY = 0; //軌跡に添うようなyの増分
int boundsX = width + baseSize; //描画領域+図形のサイズ
int boundsY = height + baseSize;//描画領域+図形のサイズ
float drawPointX = 0; //描画単位を描くx座標
float drawPointY = 0; //描画単位を描くY座標
//軌跡用の座標を結ぶ直線に添って描画単位を描画
for( int i = 0; i < pointNum-1; i++ ) {
//現在の座標と次の座標を結ぶ直線の角度を求める
angle = atan2( pointArray[i+1][1] - pointArray[i][1],
pointArray[i+1][0] - pointArray[i][0] );
//描画位置を一番始めの座標に設定
drawPointX = pointArray[i][0];
drawPointY = pointArray[i][1];
//色の配列の要素を指定する数をランダムに生成
int colorIndex = int( random( colorArray.length ) );
//軌跡に添って描画範囲内で描画単位を描画
while( -baseSize < drawPointX && drawPointX < boundsX &&
-baseSize < drawPointY && drawPointY < boundsY ) {
//baseSizeから軌跡上の移動範囲と描画サイズをランダムに設定
shapePitch = random( baseSize/4, baseSize*3/4 );
shapeSize = random( shapePitch );
//軌跡上のshapePitchに対応するxとyの増分
plusX = cos( angle ) * shapePitch;
plusY = sin( angle ) * shapePitch;
//描画色の透明度を128〜256の範囲で設定
fill( colorArray[ colorIndex ], random( 128, 204 ) );
//描画単位を描画
drawShape( drawPointX, drawPointY, shapeSize, plusX, plusY, angle );
//描画位置をxをyの増分だけ増やす
drawPointX = drawPointX + plusX;
drawPointY = drawPointY + plusY;
}
}
}
//描画単位の設定
void drawShape( float centerX, float centerY, float drawSize, float vx, float vy, float ang ) {
//メインの円弧
float yuragiX = random( -drawSize/2, drawSize/2 );
float yuragiY = random( -drawSize/2, drawSize/2 );
arc( centerX + yuragiX, centerY + yuragiY,
drawSize, drawSize, ang, ang + radians( random( 90, 270 ) ) );
//サブの円弧
float subSize = random( drawSize/2, drawSize );
arc( centerX + vx/2, centerY + vy/2,
subSize, subSize, ang, ang + radians( random( 180, 360 ) ) );
subSize = random( drawSize/2, drawSize );
float tmpRad = random( 180, 360 );
if( tmpRad > ang ) {
ang += 360;
}
arc( centerX - vx/2, centerY - vy/2,
subSize, subSize, radians( random( tmpRad ) ), ang );
//細かなストローク
if( vx > vy ) {
yuragiX = 0;
yuragiY = random( drawSize );
} else {
yuragiX = random( drawSize );
yuragiY = 0;
}
beginShape();
vertex( centerX, centerY );
bezierVertex( centerX - vx/4 + yuragiX, centerY - vy/4 + yuragiY,
centerX + vx/2 + yuragiX, centerY + vy/2 + yuragiY,
centerX + vx, centerY + vy );
bezierVertex( centerX + vx/2 - yuragiX, centerY + vy/2 - yuragiY,
centerX - vx/4 - yuragiX, centerY - vy/4 - yuragiY,
centerX, centerY );
endShape();
//小さい円弧
float splashWidth = 12;
float splashNum = random( 6 );
float splashSize = random( 3 );
for( int i = 0; i < splashNum; i++ ) {
arc( centerX + random( -splashWidth, splashWidth ),
centerY + random( -splashWidth, splashWidth ),
splashSize, splashSize, 0, radians( 180 ) );
}
}
ページの先頭へ↑
プロセス1
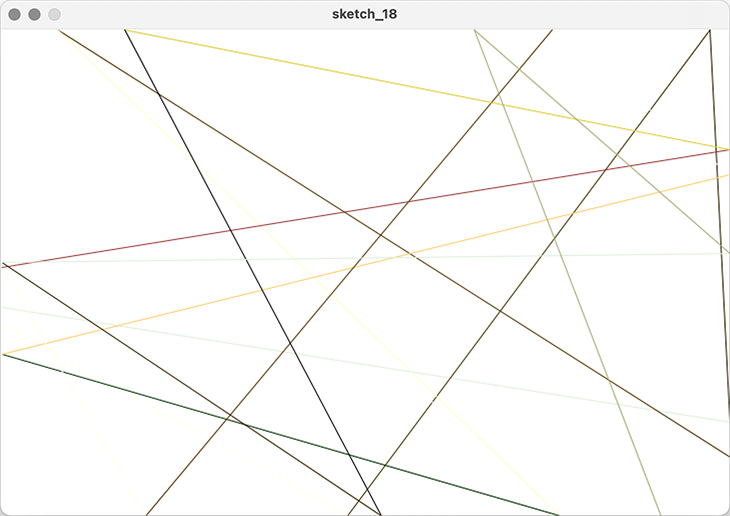
プロセス2
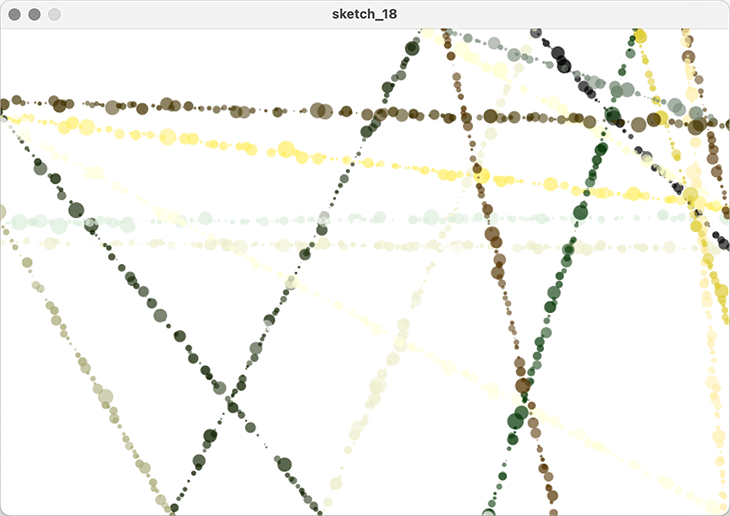
プロセス3
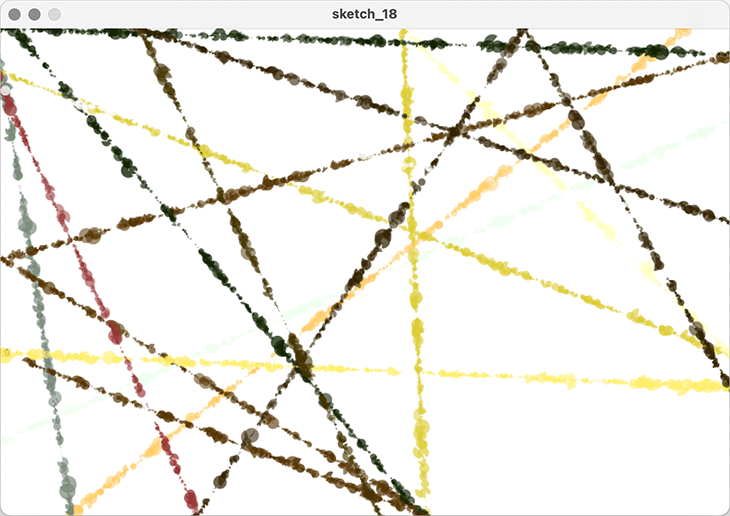
プロセス4
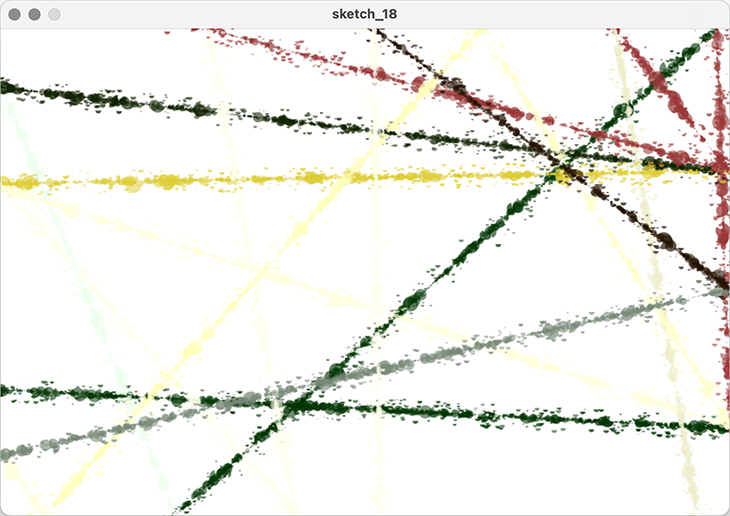
プロセス5
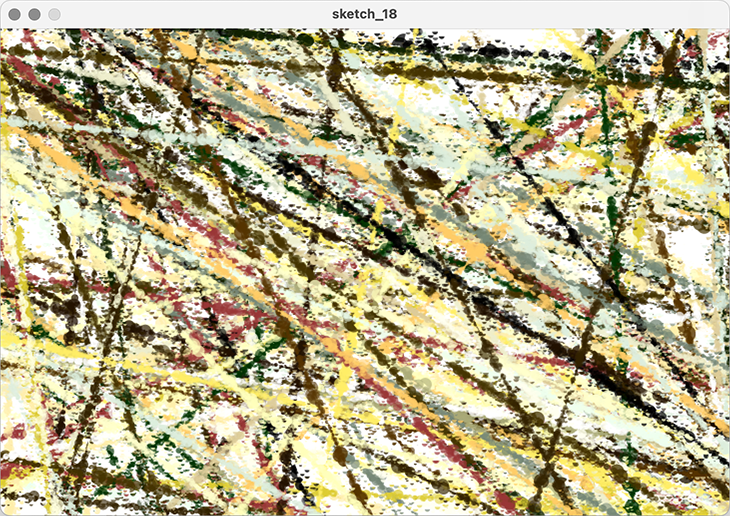
ページの先頭へ↑
< 17. 作例1